How to add the custom local font in NextJS.v14(or v13)
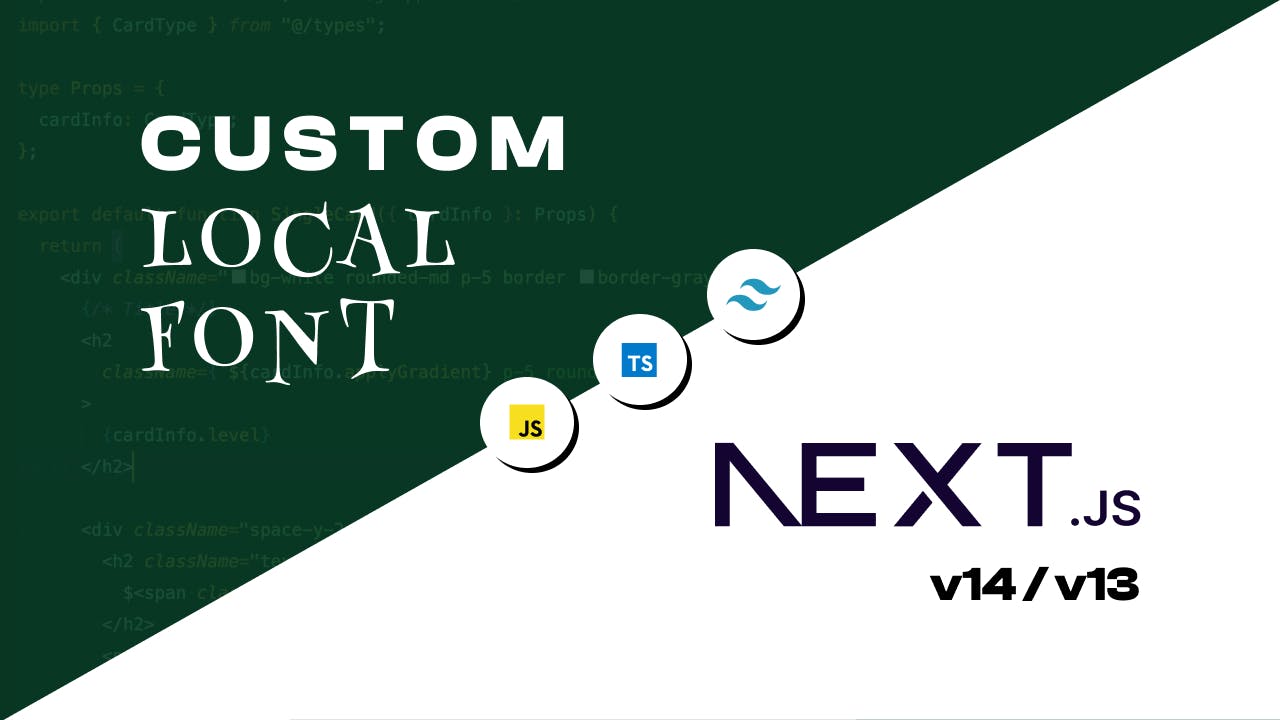
Introduction
NextJS 14 provides the @next/font/local loader add the local/external fonts on our website. Before jumping into it, let’s install the NextJS 14 first.
Even if you are using the NextJS 13, there is no such difference in the process.
NextJS Installation
Yo can use any package manager for the nextjs installation. In my case, I am using npx.
Once the installation is completed, open it in your favourite IDE (In my case, I am using VSCode.)
Adding the local fonts files in the assets folder
- Next in order to use the local fonts, we need to add those fonts in our project folder too.
- You can use any font you want. In my case, I am using the
ClashDisplay
font which you can get from my repo. Otherwise you can download this font by clicking me 🚀. - And once completed downloading, create the folder called
assets
in the root directory and inside that create the folder calledfonts
and place the downloaded font family.
It is not the compulsory pattern to follow, you can setup your folder structure as per your need.
Making use of Font
- Now we need to make use of the
next/font/local
font loader. - Before that let’s create the
utils
folder in the root directory and inside the create a file called thecustomFonts.ts
inside which will export the font family. If you want you can directly use the next/font/local, in thelayout.tsx
page. But I prefer this separate file approach as it helps to manage the code properly and clutter free. - And inside the
customFonts.tsx
orcustomFonts.jsx
the code goes like this
Note : If you are confused about what weights to give, you can checkout the weights pattern in the Tailwind Website. itself.
Explanation
- Firstly we imported the localFont from the
next/font/local
- Then we create the variable with the desired name, which we will be exporting later. You can use any name.
- And inside the localFont we passed the object containing the src and the variable key, where src key is again an object which holds the keys like path, weight, style and so on while the variable is simply the name for the font which we need later on.
- Note : If there is single font weight, we can simply pass the src with the path only, but the above pattern works fine for the font having both the single and the multiple font weights.
Adding that font in the body
Using the font without TailwindCSS
If you are not using the tailwindCSS, you can use the font easily by adding it in the globals.css
file. No need to follow other topics below this code.
Adding that font in the tailwind.config.ts
- As we are using the tailwindcss in our project. So lets add this font library in our
tailwind.config.js
, so that we can use it as a class. - Lets open the tailwindconfig file and add below code inside teh theme.
Note: the var field must match the variable value we provided in the
customFonts.tsx
file.
Using the font in our project
Finally all the setups are complete, now we can use the font buy using the classname we provided by adding the font- prefix before the frontend.
Want to add the multiple fonts, then checkout this video.
Helpful Links
Using custom fonts in NextJS
- https://nextjs.org/docs/app/-your-application/optimizing/fonts#local-fonts
- https://blog.logrocket.com/next-js-font-optimization-custom-google-fonts/
- https://stackoverflow.com/questions/74607996/how-to-add-custom-local-fonts-to-a-nextjs-13-tailwind-project